The 3D printed Implant:
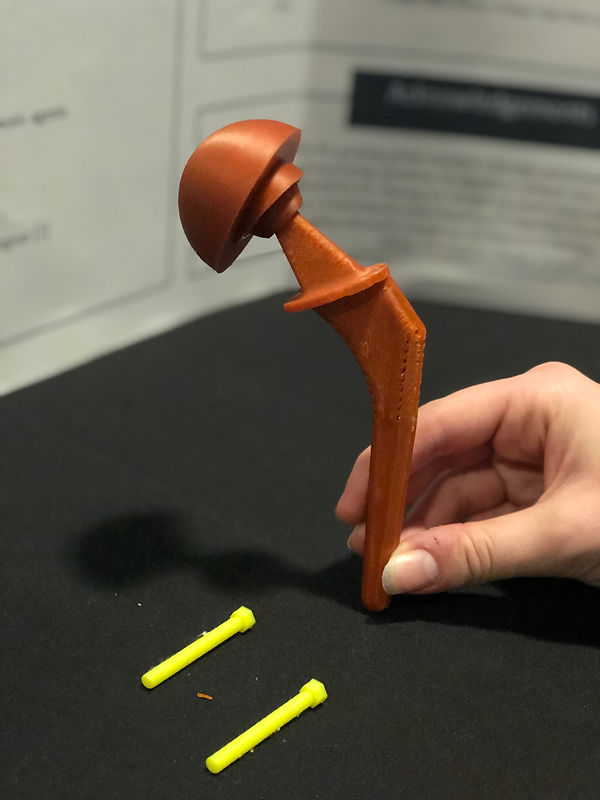
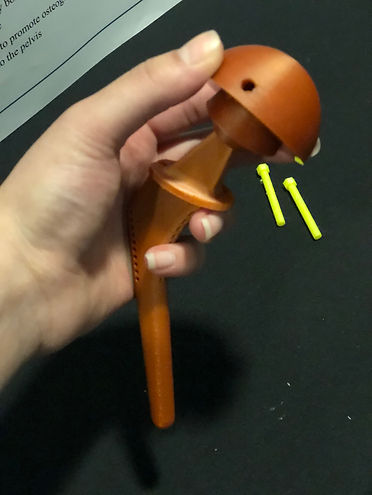
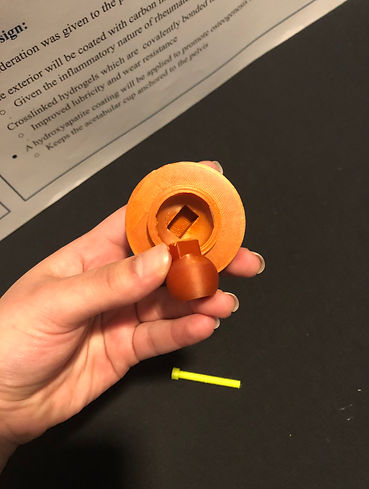
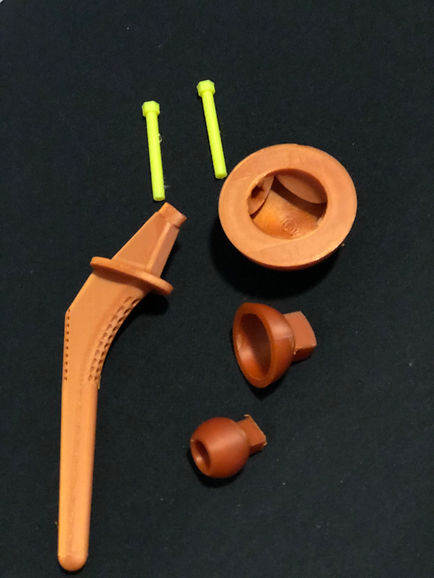
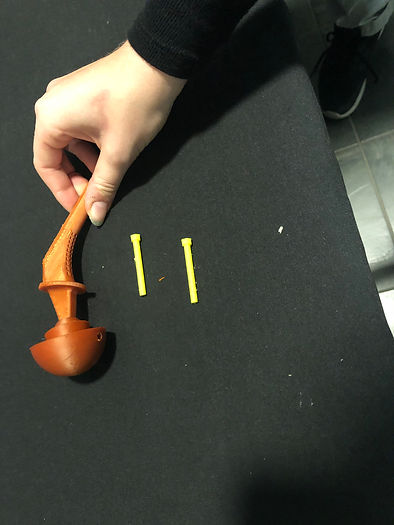

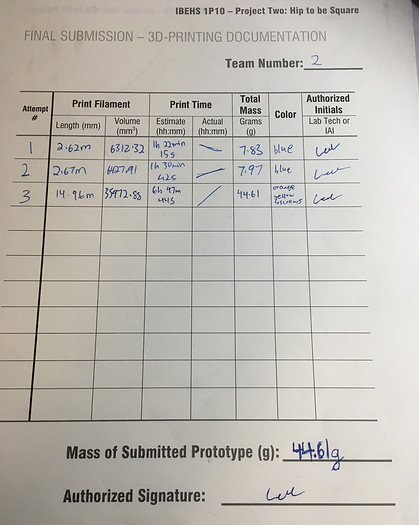
Poster board:
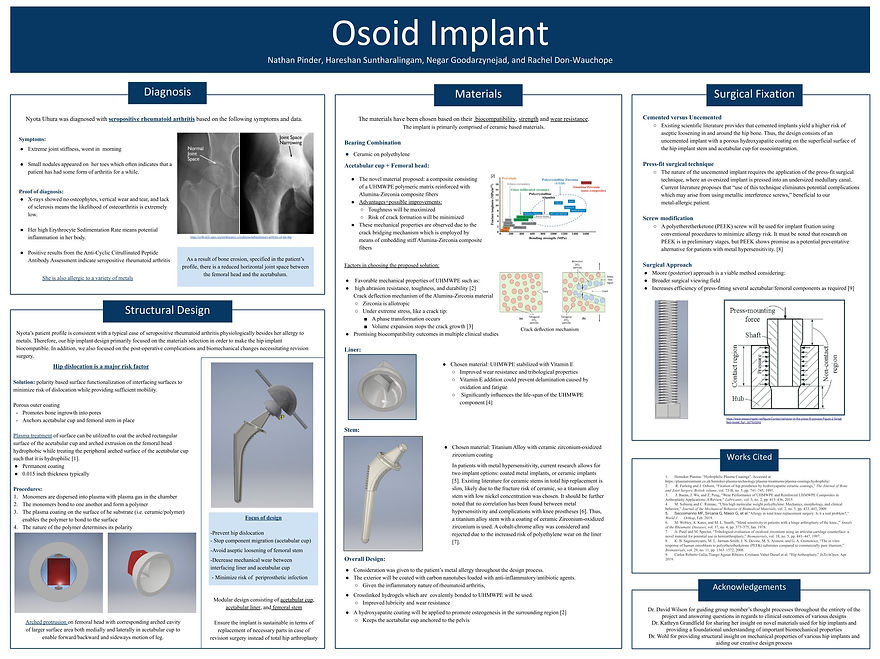
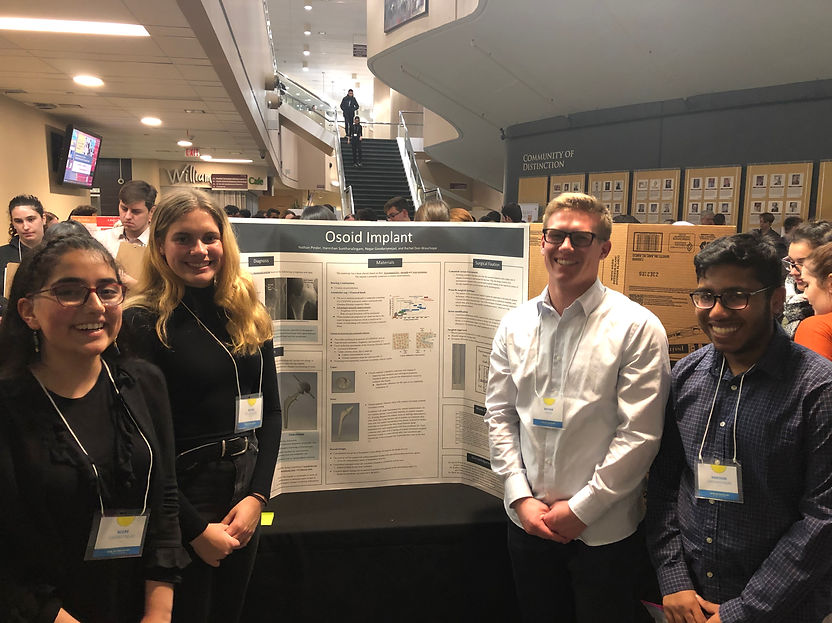
CAD File of the implant:
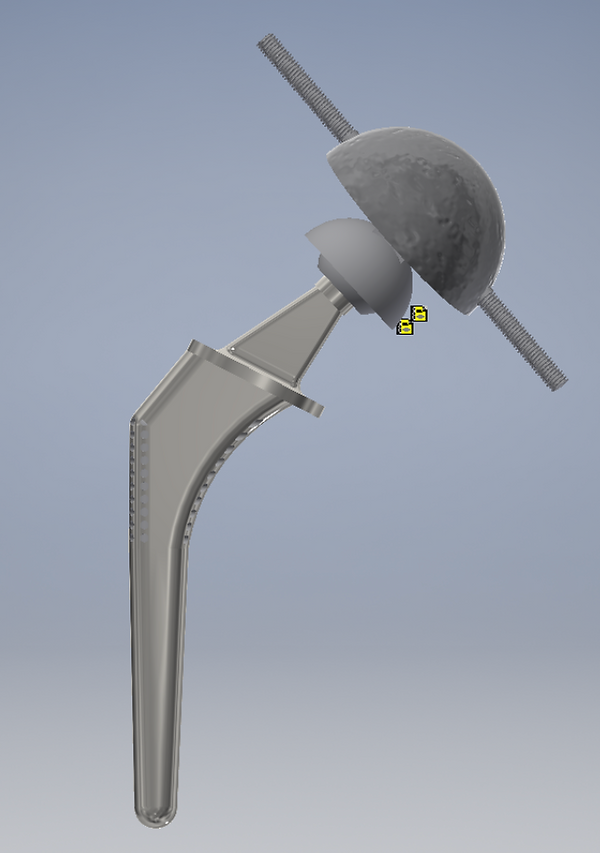
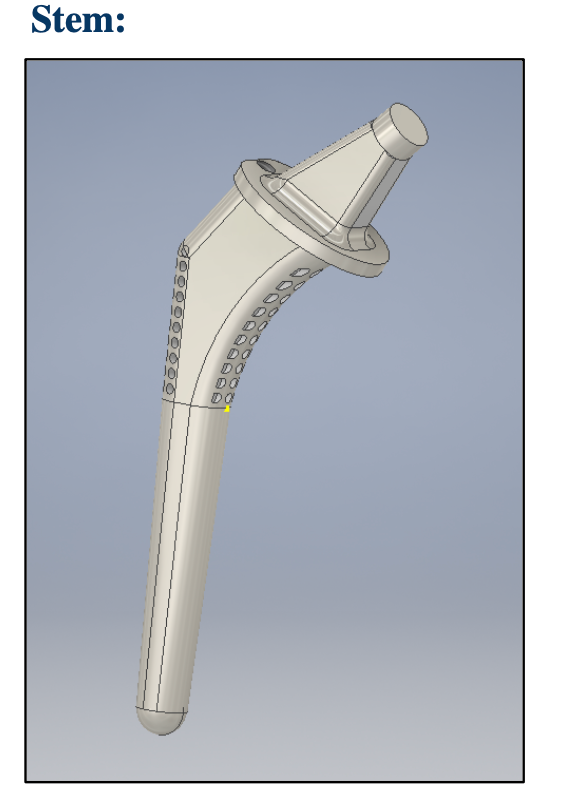
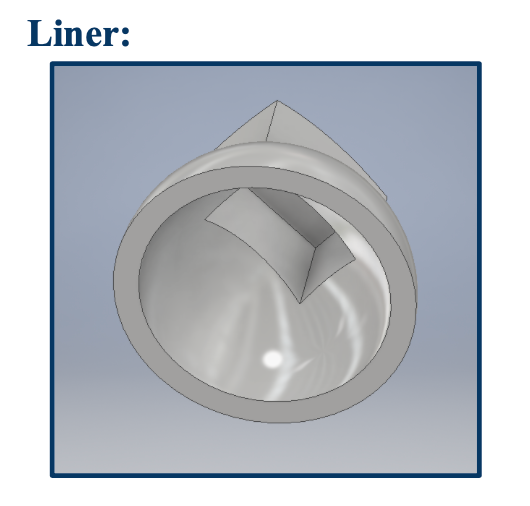
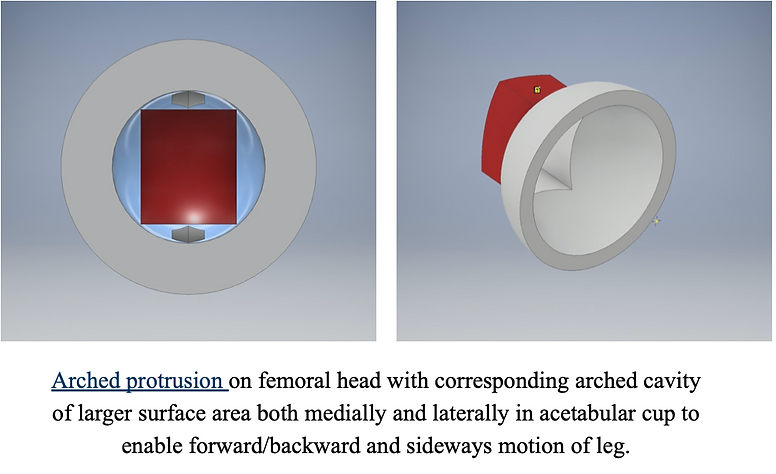
Python script:
A main function that calculates a variety of mechanical properties of the implant based on the material.
import math
import time
team_number = 2
body_weight = 54.0
outer_dia = 0
canal_diameter = 14
canal_offset = 31
ult_ten_strength = 1000
modulus_bone = 17*10**3
modulus_implant = 114*10**3
def main():
print("PROGRAM MENU")
print()
print("SUBPROGRAM 1 (1)")
print("SUBPROGRAM 2 (2)")
print("SUBPROGRAM 3 (3)")
print("EXIT (4)")
print()
choice = int(input("place choice number here: "))
if choice == 1:
subprogram1()
print()
time.sleep(1.5)
main()
if choice == 2:
subprogram2()
print()
time.sleep(1.5)
main()
if choice == 3:
subprogram3()
print()
time.sleep(1.5)
main()
if choice == 4:
exit()
def subprogram1():
# AUTHOR: Nathan Pinder (pindern)
# Subprogram 1 calculates the minimum stem diameter. An inequality is formed comparing ultimate tensile strength and applied tensile stress.
# A "while" loop cycles values of stem diameter until a value of stem_dia is no longer true for the inequality. The value is printed.
# The returned value is the minimum possible stem diameter.
stem_dia = canal_diameter
## combined loading scenario = app_ten_stress
while ult_ten_strength >= (((3.5 * body_weight * 9.81) * canal_offset) * (0.5 * stem_dia) / ((math.pi/64) * ((stem_dia)**4)) - (3.5 * body_weight * 9.81)/((math.pi/4)*(stem_dia)**2)):
stem_dia = stem_dia - 0.01
min_stem_dia = stem_dia
print()
print("SUBPROGRAM 1 RESULTS")
print()
print('body_weight =', body_weight, "kg")
print('canal_diameter =', canal_diameter, "mm")
print('ult_ten_strength =', ult_ten_strength, "MPa")
print("min_stem_dia =", round(min_stem_dia,2), "mm")
app_ten_stress = (((3.5 * body_weight * 9.81) * canal_offset) * (0.5 * stem_dia) / ((math.pi/64) * ((stem_dia)**4)) - (3.5 * body_weight * 9.81)/((math.pi/4)*(stem_dia)**2))
print("app_ten_stress = ", round(app_ten_stress,2), "MPa")
def subprogram2():
#Author: Negar Goodarzynejad (goodarzn)
#This subprogram calculates the number of cycles the implant will function before it fails
#It essentially calculates the fatigue life of the implant using the stress amplitude of the materials prposed
#The subprogram read the file, splits the data into two columns, calculates an adjusted stress amplitude from the original values using a for loop.
#Finally, the program compares each item in both stress amplitude columns and will notify the user when adjusted stress is more than stress amplitude which corrspons to failure.
#Based on the material and the data, this implant does not fail.
print()
stem_dia = float(input("input integer value of minimum stem diameter (calculated in subprogram1): "))
max_cyc_load= 10 * body_weight
min_cyc_load= -10 * body_weight
file= open('SN Data - Sample Ceramic.txt', 'r')
stress_amplitude=[]
Num_Cycles=[]
for line in file:
data= line.split()
stress_amplitude.append(float(data[0]))
Num_Cycles.append(float(data[1]))
file.close()
KN_values=[]
for item in Num_Cycles:
KN= 6 + math.log(item,10)**(team_number/30)
KN_values.append(float(KN))
Adj_stress=[]
print()
print("SUBPROGRAM 2 RESULTS")
print()
for value in KN_values:
area= (math.pi)*(stem_dia)**2
stress_max= max_cyc_load/area
stress_min= min_cyc_load/area
stressAmplitude= (stress_max-stress_min)/2
StressAmp_adj= (value*stressAmplitude)
Adj_stress.append(float(StressAmp_adj))
counter=True
for i in range(0, len(stress_amplitude)):
if stress_amplitude[i]<Adj_stress[i]:
cyclesFail= round(Num_Cycles[i],2)
stressFail= round(stress_amplitude[i],2)
print("The Number of cycles at which failure to fatigue is expected to occur is",cyclesFail )
print("The adjusted stress amplitude that corresponds to failure is",stressFail , "MPa")
counter = False
break
if counter == True:
print("Based on the given material and the stem diameter, the hip implant never fails.")
def subprogram3():
#Author: #Nathan Pinder
print()
stem_dia = float(input("input integer value of minimum stem diameter (calculated in subprogram1): "))
print()
stress_comp = (30 * body_weight * 9.81)/((math.pi/4)*(stem_dia)**2)
stress_reduc = stress_comp * ((2*modulus_bone)/(modulus_bone + modulus_implant))**(1/2)
E_ratio = (modulus_implant/modulus_bone)**(1/2)
# Same theory as SUBPROGRAM 1. The "while" function performs comp_strength <= stress_reduc and prints the first value of num_yrs that does not meet the inequality.
num_yrs = 8898
while 0.001 * (num_yrs)**2 - 3.437 * E_ratio * num_yrs + 181.72 >= stress_comp * ((2*modulus_bone)/(modulus_bone + modulus_implant))**(1/2):
num_yrs = num_yrs - 0.001
print()
print("SUBPROGRAM 3 RESULTS")
print()
yrs_fail = num_yrs
print("yrs_fail =", round(yrs_fail,2), "years")
stress_fail = 0.001 * (num_yrs)**2 - 3.437 * E_ratio * num_yrs + 181.72
print('stress_fail =', round(stress_fail,2),"MPa")
main()